Simplify Vite module imports with @-notation
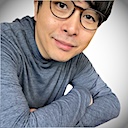
Founder and CEO
One of the subtle yet pleasant features of Next.js is its ability to import ES modules, such as React components, using path aliases. This blog post demonstrates how to achieve the same syntax sugar with Vite, a powerful and popular build tool for frontend web applications. First, consider the following simplified Vite-based project structure:
.
├── src
│ └── components
│ ├── CreateButton.tsx
│ └── DeleteButton.tsx
│
├── tsconfig.json
└── vite.config.ts
The goal is to enable module imports from anywhere in the project, as shown below, instead of using relative paths:
import CreateButton from '@components/CreateButton';
import DeleteButton from '@components/DeleteButton';
const ExampleForm = () => {
return (
<form>
<CreateButton />
<DeleteButton />
</form>
)
};
Configure resolve.alias
in vite.config.ts
In Vite, the configuration for path aliases is called resolve.alias
.
Given the project structure above, we can add resolve.alias
to vite.config.ts
as follows:
import path from 'path';
export default defineConfig({
resolve: {
alias: {
'@components': path.resolve(__dirname, 'src/components/')
}
}
});
Technically, Next.js
uses a slightly different notation with a forward-slash after the @-symbol.
If this notation is preferred, it can be replicated as follows:
resolve: {
alias: {
'@/components': path.resolve(__dirname, 'src/components/')
}
}
That's it! Vite should now be able to resolve the new alias to its actual location.
This technique can also be applied to other folders, such as: src/contexts/
.
Handling VSCode Errors
If you use VSCode, you might see an error like this after configuring Vite with path aliases:
Cannot find module '@components/CreateButton' or its corresponding type declarations.ts(2307)
This error occurs because VSCode
is not aware of the path aliases configured in Vite.
It can be resolved by configuring the paths
option in the compilerOptions
section of either tsconfig.json
or jsconfig.json
to match Vite's path aliases.
{
"compilerOptions": {
"paths": {
"@components/*": ["./src/components/*"]
}
}
}
Enhancing Developer Morale
This blog post demonstrated how using Vite's path aliases can add syntactic sugar to import statements in a web frontend project.
Because of the popularity of React
and its component-based architecture, import statements are highly visible in modern web frontend projects.
These subtle touches can enhance developer morale and make the project more enjoyable to work on.
Happy coding, and enjoy the simplified syntax!